ExtendScript
My favorite Adobe applications are all scriptable. They use a scripting language called ExtendScript. I think it’s pure and compliant JavaScript (aka EcmaScript)… if it has any deviations, it’s in some subtle fashion that hasn’t affected me.
One thing you frequently need in a script is a means to gather input choices, so the script can be flexible. I rolled something up that handles this for many simple situations. I call it the Omino Dialog Maker. It’s a software library that lets you bring up an input dialog with just a couple of lines of code. It is mostly about 2 years old now, works pretty well.
Omino Dialog Maker
Here’s a simple example of a dialog made with the Omino Dialog Maker.
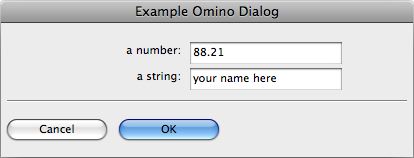
And here’s the code to produce it:
#include "ominoDialogMaker.jsx"
var omd = newOminoDialog("Example Omino Dialog");
omd.number("a number","n",88.21);
omd.string("a string","s","your name here");
var result = omd.run();
if(result == null)
alert("Cancelled\nYou clicked \"cancel\".");
else
alert("n is " + result.n + ", and s is \"" + result.s + "\"");
That’s pretty handy, isn’t it? As you can see, omd.run()
populates a result variable with the contents of the dialog.
I admit it: tt’s not the best possible dialog and layout. It’s a little clunky looking. But it gets the job done, lets me type in a few numbers and set meat of the script to work.
More UI Elements
Here’s a dialog which shows the various UI elements supported by Omino Dialogs:
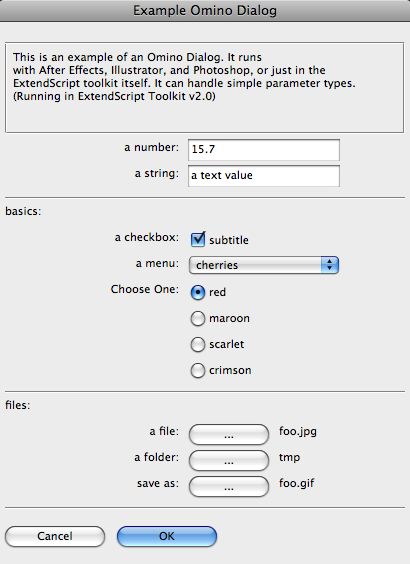
And, here’s the code which produced it:
#include "ominoDialogMaker.jsx"
function go()
{
// setting up a dialog is easy. you can add entries for basic types of
// string and number, and checkbox and radio buttons, and the
// dialog is presented reasonably, with very little work.
var omd = newOminoDialog("Example Omino Dialog");
omd.boxedText(5,"This is an example of an Omino Dialog. It runs \n"
+ "with After Effects, Illustrator, and Photoshop, or just in the\n"
+ "ExtendScript toolkit itself. It can handle simple parameter types."
+ " (Running in " + app.name + " v" + app.version + ")"
);
omd.number("a number","n",15.7);
omd.string("a string","s","a text value");
omd.separator();
omd.sectionLabel("basics");
omd.checkbox("a checkbox","x",true,"subtitle");
omd.menu("a menu","menu","cherries",["apples","bananas","cherries","dates","figs"]);
omd.radioButtons("Choose One","r","red",["red","maroon","scarlet","crimson"]);
omd.separator();
omd.sectionLabel("files");
omd.openFile("a file","jpgFileName","foo.jpg","Choose a JPEG File",".jpg");
omd.selectFolder("a folder","folderName","/tmp","Choose a folder");
omd.saveFile("save as","gifFileName","foo.gif","Save as GIF",".gif");s
// when we "run" the dialog, we get back a result variable.
// if you hit CANCEL, then the result is null.
// if you hit OK, then the values are populated into the result.
// note: you can kill photoshop by running the dialog from ExtendScript toolkit, then halting
// the script with the dialog still up.
var result = omd.run();
if(result == null)
alert("Cancelled\nYou clicked \"cancel\".");
else
{
var s = "result from dialog:\n";
for(var f in result)
s += " " + f + " := " + result[f] + "\n";
alert(s);
}
}
go();
If you’ve read this far, you’re hopefully asking, “Gee, where can I get the actual library?” Here’s the code, shown directly out of my source code repository. Download with the download button.
Â
Trivia: UI Differences Across Adobe Applications
Omino Dialog Maker can be used for scripts in After Effects, Photoshop, and Illustrator, at least, and possibly other Adobe applications. I have noticed that the dialogs look slightly different in each of these apps! Not critically… For reference, here are screen shots from several.
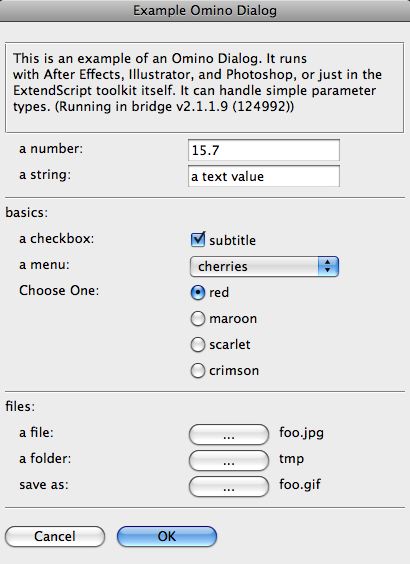
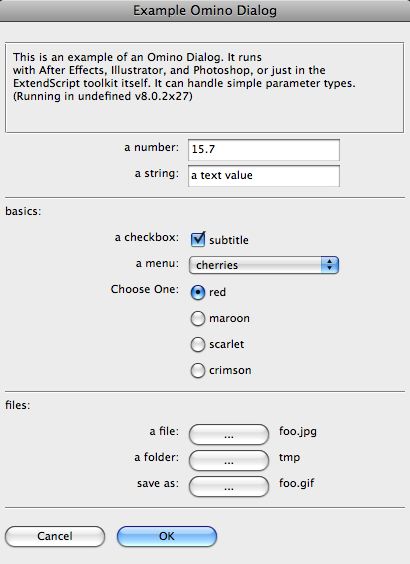
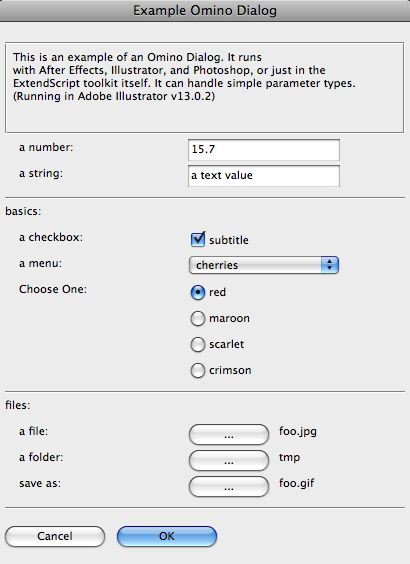
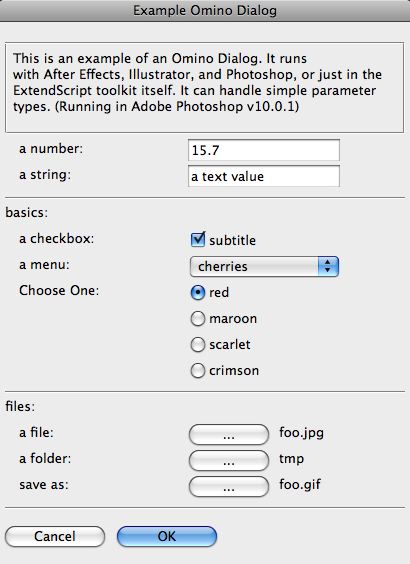